Angular SEO friendly coding practices
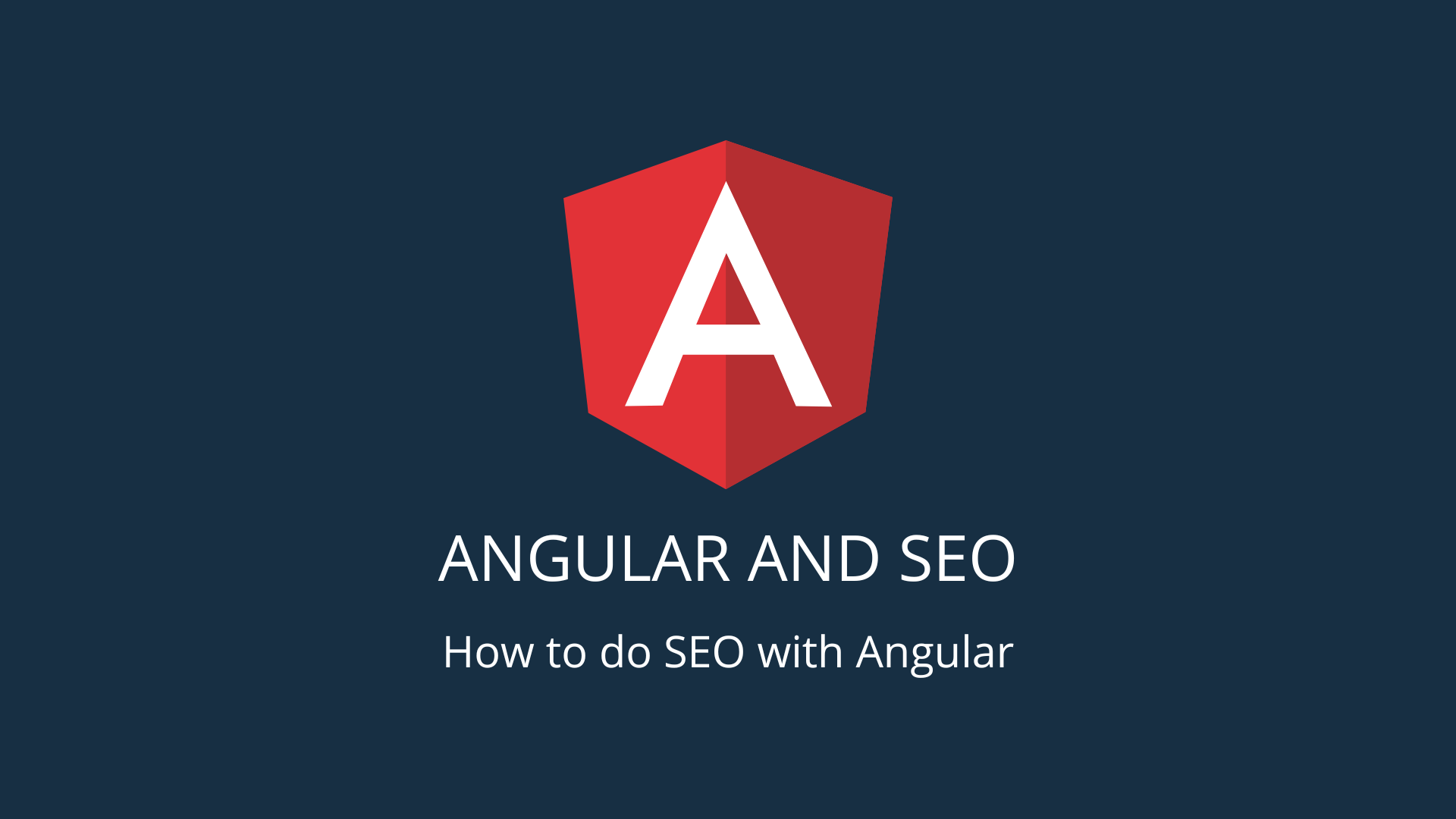
If you are developing a website or application with Angular chances are you want it to rank well in the search engines. As you might have read already, this comes with some challenges. Angular is a well-known front-end framework widely used to build single-page applications, better known as SPA's. They are well known for their smooth and instant page transitions and great user experience. But there are some drawbacks especially when it comes to SEO performance.
Table of content:
Angular SEO friendly coding practices
Improve Angular SEO with prerendering
Adding Meta tags for Angular SEO
Angular SEO challenges
When building SEO friendly applications with Angular you run into some challenges. These challenges arise from the fact that (by default) you are building a SPA application with Angular. When you build a SPA application it will generate some CSS files, JS bundles, and one HTML page (index.html). On this HTML page, we find little to no content, only some script to load our CSS and JS files. The JS files will then be processed by the browser to render the pages of your application. This is great for some purposes, but crawlers from search engines and social media websites might have trouble indexing your pages if they only receive one page and some JavaScript code.
There is some good news, the crawlers of Google are getting quite good at indexing JavaScript content, but it still does not compare to the indexing of well-structured HTML files with all the content contained in them.
Another drawback that SPA applications bring on the SEO front is that they have a slow initial page load. With SPA applications you load a full JavaScript module upfront and then navigate seamlessly through the rest of the application's pages. But the loading of this JavaScript module costs a lot more time than loading an HTML page resulting in a slow initial page load. Initial page loads are a big factor when optimizing for SEO, so this is an attention point as well when building SEO friendly Angular applications. If you are deadset on a SPA application you can try to keep your initial bundle as small as possible by creating a lightweight app module and lazy loading all other modules after a redirect for example.
Angular SEO friendly coding practices
Now that you have a better understanding of the problems you face when building SEO friendly Angular applications, let's have a look at how we can solve them! A brief overview of what you can do to improve SEO for your Angular application:
Angular Universal and server-side rendering: We can improve the SEO performance of our Angular application drastically by using Angular Universal and serve-side rendering
Prerender your Angular application: Prerendering your Angular application is another great way to improve SEO for your Angular application
Add Title and Meta tags dynamically: Adding a title and meta tags on each HTML page is a crucial part of on-page SEO. With the Meta and Title service of Angular, you can add these tags dynamically so every page has its own tags
Add a sitemap: A sitemap is an XML file that lists all the URLs of your website. This helps search engines understand the structure of your website and makes it easier for them to index your pages
Robots.txt: Robots.txt is a file that tells search engines which pages or sections of your website should not be indexed
Improve Angular SEO with SSR
When you're talking about SEO friendly Angular applications one of the first things to look at is the way you render the content. As previously explained with a SPA application the content is rendered with JavaScript code in the browser in a single HTML document. For SEO purposes it would be better if each page of your application would have a completely rendered HTML page with all the content of the page in the HTML document. This way we can ship this HTML page instead of some javascript code to the browser making it much easier for search engines to index the pages. Not only will this make it easier for search engines to index your pages, but it will also improve your initial page loads again boosting your SEO performance.
So how is all of this achieved you ask?!
To achieve this you want to enable server-side rendering, meaning you have a server that will listen for requests on your page URLs and when it receives a request the server will render the HTML page on the server and send an HTML page to the browser. If this all sounds very complex don't worry, Angular has got you covered! Angular comes with a package called Angular Universal, and it's your best friend when building SEO friendly Angular applications.
With Angular Universal you can transform your application into a server-side rendered application just by running one command:
ng add @nguniversal/express-engine
This command will generate an express.js server for you and add some configurations and node js scripts to your Angular application so it can be rendered on the server. If you want a full how-to on Angular Universal and Server side rendering you can read this article: Angular Universal Server Side Rendering (SSR) and how to use it
After you enabled SSR with Angular Universal your pages will be rendered on the server making your pages easy to index for search engines and it will improve your initial page loads giving you another SEO boost. Another cool thing about Angular Universal is that it gives you the best of both worlds. The pages are rendered on the server, but after the page is loaded, it will swap the website for the SPA application giving you the instant navigation feature of the SPA as well.
Improve Angular SEO with prerendering
Another way to improve your Angular SEO is by prerendering your pages. In the previous example (SSR) the pages were rendered on the server each time the page is requested. With prerendering, you render the HTML pages during your build process. This will only work for static content but can even further improve your page loads and the control you have over the rendered HTML pages. It can also help to make a dent in your hosting costs as prerendered applications can be hosted as a static website whereas an SSR application needs hoasting for your server.
Prerendering your Angular application can also be done using Angular Universal or another popular alternative is to use Scully a library for prerendering Angular applications. If you want a full how-to on prerendering with Angular Universal you can read this article:
Angular Static Site Generation - A full guide
Adding Meta tags for Angular SEO
When you are building a website with Angular and want to make sure it's optimized for search engines, one of the most important things to do is to use meta tags properly. Wheater you are building a SPA, SSR, or SSG Angular application proper meta tags are crucial for on-page SEO.
First things first: What are meta tags?
In simple terms, meta tags are snippets of code that provide information about a web page to search engines. They're placed in the head section of the HTML code and are invisible to website visitors. Some examples of meta tags include the keywords meta tag, which tells search engines the keywords of the page, and the description tag, which gives a brief summary of what the page is about. The title of the page is added with a title tag, similar to the meta tag this one also needs to be added dynamically to each page.
Meta tags play a critical role in how search engines understand and rank your website. When search engines crawl your website, they use the information in the meta tags to determine what your pages are about and how relevant they are to a user's search query. If you have well-written meta tags, it's more likely that your website will show up higher in search engine results, which means more visibility and traffic for you.
Adding meta tags in the HTML templates of your components does not work. Meta tags that are shared among all pages can be added to the index.html at the root of your Angular project. Some examples of shared meta tags might be:
<metaname="viewport"content="width=device-width, initial-scale=1">
<metaname="google-site-verification"content="some-verification-key">
<metaname="robots"content="index, follow">
<metaproperty="og:image:width"content="300">
<metaproperty="og:image:height"content="300">
Other page-specific meta tags and the title tag can be added with the Meta and Title service. Let's first have a look at how you can use the Meta service from @angular/platform-browser to add meta tags to the pages of your Angular application:
As you can see in the example you import the Meta
service from the @angular/platform-browsers
;
t
hen inject it with dependency injection in the constructor of the component class. Now you can use the addTag()
method to add a meta tag to the head of the HTML document. If you place the addTag methods in the ngOnInit it will work for a SPA and SSR but not for a prerendered application, so best to just declare them in the constructor.
Adding the title tag to a page is very similar. You import the Title service also from @angular/platform-browsers and again inject it with dependency injection and use it in the constructor like this:
Here is a list of useful meta tags to add for better SEO and social media indexing and shareability.
<meta name="twitter:description" content="">
<meta name="twitter:title" content="">
<meta name="twitter:image" content="">
<meta name="og:type" content="">
<meta name="og:description" content="">
<meta name="og:title" content="">
<meta name="og:image:secure_url" content="">
<meta name="og:image" content="">
<meta name="keywords" content="">
<meta name="description" content="">
Add a sitemap.xml and robots.txt for SEO
You are almost there, just some final touches to improve your Angular SEO. The last thing we will do in this blog post, adding a robots.txt and a sitempa.xml. Robots.txt is a file that tells search engines which pages or sections of your website should not be indexed. A sitemap is an XML file that lists all the URLs of your website. This helps search engines understand the structure of your website and makes it easier for them to index your pages.
Both files can be added to the src of your Angular project.

The content of the robots.txt should look something like this:
User-agent: Googlebot
Disallow: /nogooglebot/
User-agent: *
Allow: /
Sitemap: https://example.com/sitemap.xml
The content of the sitemap should look like this:
Conclusion
Your Angular application may not be SEO optimized from the get-go, but with Angular Universal and the Meta and Title service you can make SEO friendly Angular application! With not much effort you can turn your Angular application into a server-side rendered or prerendered application with HTML pages for the search engines to index. With the meta en title service, you can add dynamic meta and title tags to your pages so search engines can index your pages with ease.